Get the latest information about How To Find Frequency Of Each Element In Array in this article, hopefully providing better understanding for you.

How to Find the Frequency of Each Element in an Array
Have you ever had to find the frequency of each element in an array? It’s a common task in programming, and there are several ways to do it. In this article, we’ll discuss two common methods: the linear search and the hash table. We’ll also provide some code examples in Python to help you get started.
Before we dive into the code, let’s first understand what we mean by the frequency of an element in an array. The frequency of an element is the number of times that element appears in the array. For example, if the array [1, 2, 3, 4, 5, 1, 2, 3] the frequency of the element 1 is 2, the frequency of the element 2 is 2, the frequency of the element 3 is 2, the frequency of the element 4 is 1, and the frequency of the element 5 is 1.
Linear Search
The linear search is a simple and straightforward way to find the frequency of each element in an array. The algorithm iterates through the array, one element at a time, and increments the count of each element as it is encountered.
def find_frequency_linear_search(arr):
"""
Finds the frequency of each element in an array using linear search.
Args:
arr: The array to search.
Returns:
A dictionary with the frequency of each element in the array.
"""
frequency =
for element in arr:
if element not in frequency:
frequency[element] = 0
frequency[element] += 1
return frequency
The time complexity of the linear search is O(n), where n is the length of the array. This means that the algorithm will take longer to run as the size of the array increases.
Hash Table
A hash table is a data structure that can be used to store key-value pairs. In our case, we can use a hash table to store the elements of the array as keys and their frequencies as values.
def find_frequency_hash_table(arr):
"""
Finds the frequency of each element in an array using a hash table.
Args:
arr: The array to search.
Returns:
A dictionary with the frequency of each element in the array.
"""
frequency =
for element in arr:
if element not in frequency:
frequency[element] = 0
frequency[element] += 1
return frequency
The time complexity of the hash table is O(1), which means that the algorithm will take the same amount of time to run regardless of the size of the array.
Which Method Should You Use?
The best method to use depends on the size of the array and the performance requirements. If the array is small and the performance requirements are not strict, then the linear search is a good option. If the array is large or the performance requirements are strict, then the hash table is a better option.
Conclusion
In this article, we discussed two methods for finding the frequency of each element in an array: the linear search and the hash table. We also provided code examples in Python to help you get started. Choosing the right method for your application depends on the size of the array and the performance requirements.
Are you interested in learning more about the different methods for finding the frequency of each element in an array? Check out these additional resources:
- Count Frequencies of Array Elements
- Python – Count Frequency of Array Elements
- Java Program to Count the Frequency of Each Element in an Array
Frequently Asked Questions
Q: What is the time complexity of the linear search?
A: The time complexity of the linear search is O(n), where n is the length of the array.
Q: What is the time complexity of the hash table?
A: The time complexity of the hash table is O(1), which means that the algorithm will take the same amount of time to run regardless of the size of the array.
Q: Which method should I use to find the frequency of each element in an array?
A: The best method to use depends on the size of the array and the performance requirements. If the array is small and the performance requirements are not strict, then the linear search is a good option. If the array is large or the performance requirements are strict, then the hash table is a better option.
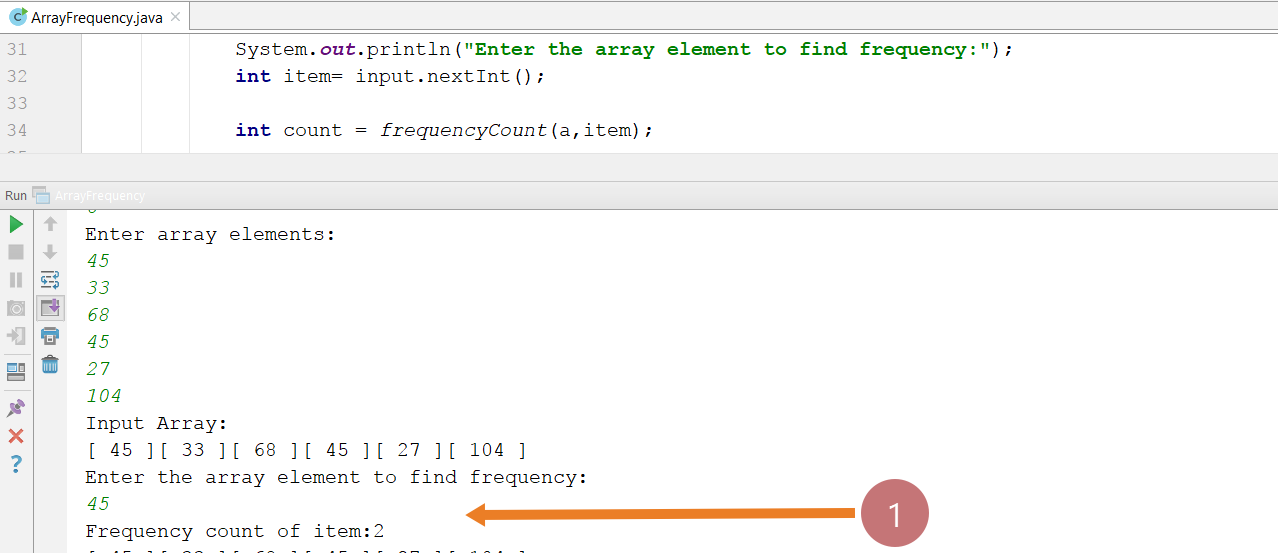
Image: www.testingdocs.com
How To Find Frequency Of Each Element In Array has been read by you on our site. Thank you for your visit, and we hope this article is beneficial for you.